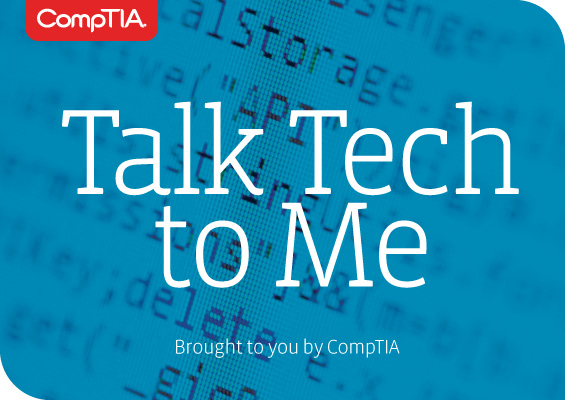
It is easy to write a script today and know exactly what it should do and how it works. But like most people, I am busy, and over time, I forget the details of what the script does and how it does it. That’s why it’s important to add documentation to your scripts while you are working on them. It can also help you when you’re looking to run other scripts – documentation in the help file can help you understand what the script is expected to do. Keep reading for tips on how to add help and parameters to your scripts to make them more robust by adding common parameters.
In my last article on how to write better PowerShell scripts, we covered using Try/Catch blocks, using special output formatting and when an error should stop a script versus just notifying you that something didn’t work as intended. In this article, we’ll be discussing comment-based help and something called CmdletBinding.
Let’s face it, we are all busy and things get crazy fast. Like most people, I do things today, but may not need to do them again for weeks or months. Adding help information to scripts about what they do, how they do it and the expected output helps me remember what I did. Now, yes, you can open up your scripts and walk the lines of code again to figure out what it does, but it’s definitely easier to add a help block at the top that gives a quick overview.
NOTE: If you don’t trust the source of a script, or found one on the internet, it is never a good idea to just run it. Always review untrusted scripts.
Comment-based Help
When I send the path of my script to “Get-Help” it will show me the basic parameter information, but not much else. The script I’m going to be using will get a file, process the data in the file and then do something on another computer. But at this point, the script does not provide much information when running “Get-Help C:\Temp\BigScript.ps1”.
Let’s change that by adding comment-based help at the top of the script.
The comment-based help appears at the top of your script. In a function, which is similar to a script in the way that you build it, the comment-based help can be at the beginning of the function body, at the end of the function body or before the Function keyword. This article won’t go into detail about functions, but keep in mind that they can also have comment-based help in three different locations and only one location in a script.
Looking at our example above, when I run “Get-Help” on my “BigScript.ps1”, you will see this output.
While this is good, it doesn’t show me the details or all the extras I put into the comment-based help. That is a feature of the “Get-Help” Cmdlet. Adding the parameter “-Full” to “Get-Help” shows all the details of my comment-based help. To learn more about “Get-Help”, you can have PowerShell show you the help file by typing “Get-Help Get-Help -Full”.
Now, I’m going to add some useful information to my help block. This is the area where you want to add as much information about your script as you can. I try to write the help description as if I am giving the script to someone who doesn’t know much about PowerShell so they can run the “Get-Help C:\Temp\BigScript.ps1 -Full” commands to see what it does. I also try to give plenty of examples and describe what the person running the script should expect to happen. Below is the help section of a script I wrote to create shared mailboxes in Exchange Online.
What you do not see in the comment-based help is any information about parameters. I like to add comments above my parameters in the param block, which I’ll talk about next time. If I give someone this script and they run the full help, they will be able to see how the script works and the expected parameters.
Another great feature of comment-based help, is adding Links to your scripts. In my example, the first “Link” in the comment-based help points to CompTIA’s website, but this can point to any webpage you want: your company homepage, the internal IT homepage, a Git page showing more details about the script or any relevant link that can provide more information. If the person running the script types “Get-Help C:\Temp\BigScript.ps1 -Online”, they will be directed to the webpage declared in the first Link in comment-based help.
CmdletBinding
Under the comment-based help section, you should add CmdletBinding and a parameter block to your scripts. By using CmdletBinding, you add something called the common parameters to your scripts and functions. According to the help file for “about_Functions_CmdletBindingAttribute”, CmdletBinding “makes [scripts and functions] operate like compiled Cmdlets that are written in C#, and it provides access to features of Cmdlets.”
In the next example, the use of “[CmdletBinding()]” on the first uncommented line gives access to the following parameters in your script: Debug, ErrorAction, ErrorVariable, InformationAction, InformationVariable, OutVariable, OutBuffer, PipelineVariable, Verbose, WarningAction and WarningVariable. So now when I use the parameter “-Verbose”, my output shows the comment I wanted in my “Write-Verbose” Cmdlet.
I can also automatically add more features to my script inside the CmdletBinding parenthesis. The full syntax for CmdletBinding is shown in the next example.
The “about Functions CmdletBindingAttribute” page from Microsoft lists details about all the options available. I think my favorite CmdletBinding attribute is “SupportsShouldProcess” followed by “DefaultParameterSetName”. “SupportsShouldProcess” adds the parameters “Confirm” and “WhatIf” to the script or function. Adding either or both of these switches causes all the Cmdlets within your script that also support these switches to automatically append the same switch to them.
In the example above, the verbose statement will run each time because the Try/Catch block only stops processing if there is a terminating event.
Lastly, let’s look at the “DefaultParameterSetName” attribute. This gives your scripts and functions the ability to do specific actions based on the parameters provided.
Notice how each parameter I am using in the param block gets its own line in the Syntax output? In other words, I can only use one parameter at a time. But what if I wanted to use a certain parameter in all of my parameter sets? I could either not include the attribute “ParameterSetName” in the parameter or declare which parameter sets the parameter belongs to.
In the example above, because I didn’t declare which parameter set “Delta” belongs to, by default, the script uses it in all three parameter sets. And because I didn’t declare that it should be mandatory, it is optional for all three parameter sets. On the other hand, the parameter “Echo” is not available in the default parameter set, optional in parameter set two and required in the third parameter set.
In my next post, I’ll go into the details of using parameters and adding the help information for parameters in the param block versus the comment-based help.
CompTIA performance-based certifications validate IT skills from the help desk to top security professionals. Learn more about CompTIA certifications.