Python is a common and popular open source programming language, and it's one of the best ways to begin learning programming. Python is cross-platform, so you can probably use whatever computer system is available to you, whether it be Linux, macOS or Windows.
Programming languages are formalized ways for developers to create applications to run on computers. Most programming languages are text-based, including Python. With Python, a developer writes code, the code is compiled (within the Python instance and hidden from the user) and interpreted on the system.
Python was developed by Guido van Rossum in the late 1980s, with version 2.0 released in 2000. Major version 3.0 was released in 2008 but is not backward compatible to 2.0, causing a variety of compatibility issues that still plague Python development today. While Python 2.7 is still widely used, it is no longer officially in development or supported. Most new implementations use the 3.0 major version.
Note: Like many software applications, Python uses a numeric scheme to describe version updates. For example, the 3.10.9 version is major version 3, minor version 10 and micro version 9. When getting started, install the latest stable version.
Python gets its name from the popular British comedy troupe Monty Python, and the language includes various references to the group.
Python Key Features
Python is characterized by being relatively simple compared to many other programming languages. Don't be fooled, however; Python is powerful and found in many essential applications. One aspect of Python that lends it simplicity is that it is a high-level language, meaning that it is human-readable. Low-level languages are easier for the machine to comprehend but more difficult for developers to read and understand. Python's simplicity means that its syntax and values are placed logically and formatted using indentions that make it easier to understand blocks of code.
Python is an object-oriented language. Developers define classes (items containing one or more attributes) and then specify instances of the class in the form of objects. The objects are then manipulated.
You've heard the phrase work smarter, not harder. Python relies on libraries to satisfy this goal. Python libraries are bundles of code written to address standard or common programming needs. They make the Python developer's job easier by providing prewritten code for many standard solutions without the developer having to rewrite it. For example, can you imagine how many existing programs might need to conduct basic mathematical computations, such as addition or subtraction? Instead of each developer worldwide having to recreate the code to do the math, they can reference the NumPy (Numerical Python) library, which contains the code necessary to solve basic math problems. The library is available to any developer whose code has mathematical calculations.
There are many available libraries. Here are a few common ones:
- Pygame: A set of modules for game development, including video and sound libraries
- NumPy: A set of modules for simple and complex math functions often used with machine learning, other libraries often use NumPy
- PyScript: A framework for creating browser-based applications using HTML, providing a front-end Python solution (where Python is normally used for back-end applications)
- Matplotlib: A set of modules for plotting numerical data for data analysis and presentation
- Pandas: A library for data analysis and manipulation
- Beautiful Soup: A library for gathering and analyzing information from the web (web scraping) via HTTP
- Requests: A library for generating HTTP requests, usually as part of a larger web scraping project
At this level, the terms library and module can be used interchangeably.
Python Integrated Development Environments (IDEs)
Many developers use dedicated programs to work with their chosen programming languages. These programs are called integrated development environments (IDEs). There are many IDEs available for Python developers.
IDEs tend to provide the following functionality:
- Editors with syntax checking specific to one or more languages
- Build tools
- Debugging tools
- Source control
A few Python IDE examples follow below. These are particularly suited to newer Python users.
PyCharm
One popular IDE is PyCharm, which is user-friendly and allows for many useful plugins. PyCharm has multiple versions, each with its own licensing mechanism. The free version is plenty powerful for those new to Python development. PyCharm is the standard by which other Python IDEs are measured.
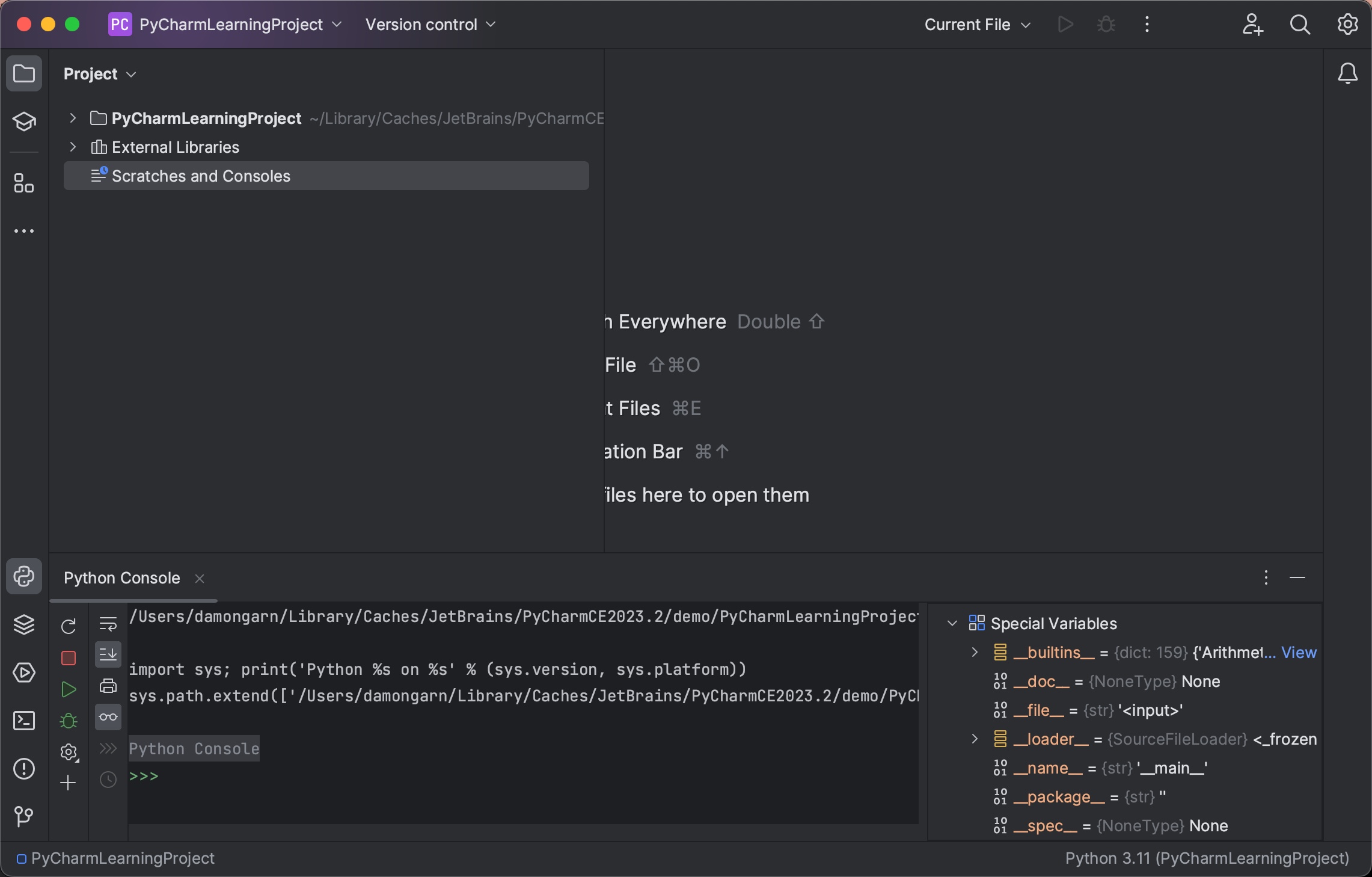
Figure 1: The PyCharm IDE
IDLE
Another common Python IDE is IDLE, or the Integrated Development and Learning Environment (this is also a reference to Monty Python's Eric Idle). IDLE is included with standard installations of Python on Linux, macOS and Windows. It is simple to use but not as full-featured as other options.
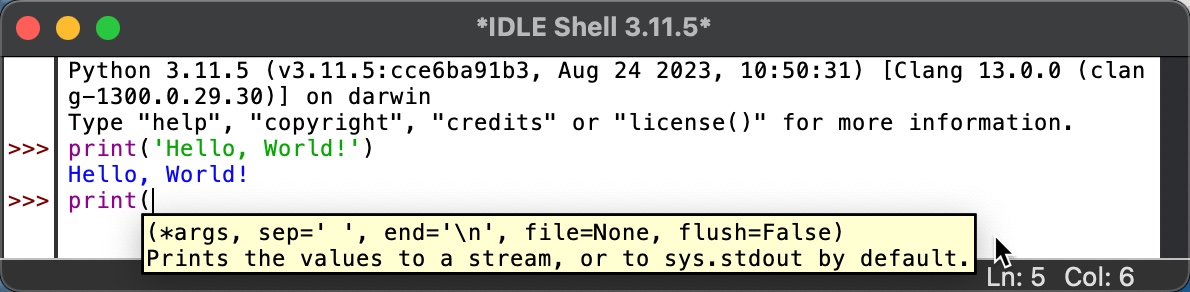
Figure 2: The IDLE IDE; note the prompt in yellow for likely syntax.
Thonny
Thonny includes Python in its installation. Once you download Thonny for your platform (Linux, macOS or Windows), you're ready to start. Thonny provides simple explanations for code, including expressions, functions and variables. Like other IDEs, it also includes syntax checking and debugging. Thonny is particularly friendly to Raspberry Pi users.
Text Editors
One doesn't need to use a dedicated IDE, though they tend to make the programming process easier. Any text editor will do, though features like syntax highlighting and code formatting are very helpful. Editors such as Vim and Emacs are easily extended to support Python and may provide enough functionality for basic tasks. Both editors are extensible to provide greater Python functionality.
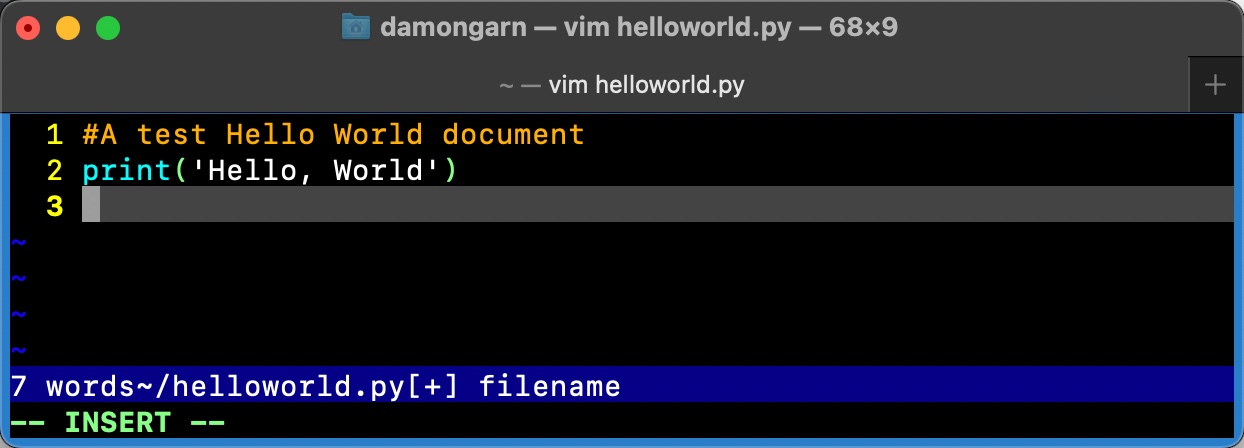
Figure 3: Using Vim to write Python code, note that many Vim versions automatically provide syntax checking
When to Use Python
So, when might you want to learn and use Python? There are several instances where Python might be a great choice:
- You are an experienced developer with a different programming language, such as Java or C++, and you want to expand your skills or make yourself more employable.
- You are new to software development and looking for an easy language to start with but one that includes plenty of career opportunities across many fields.
- You are a developer looking for a general-purpose programming language to address specific business needs, especially in the science, data science or machine learning fields.
- You are curious about how application development works and want to try a few simple coding projects.
- You are a teacher or other educator looking for a good programming language to introduce your students to the world of software development.
Python Project Examples
Organizations might look to Python to address specific business needs. Here are a few common examples where Python might contribute to addressing business processes:
- Data science, including visualization and analysis
- Machine learning solutions
- Website and web app projects
- Software development projects
- Automation solutions
- General tasks
Because Python is a non-specialized, general programming language, it appeals to many industries and is found in countless development projects.
One of the best ways to get started with Python and understand what it's good for is to do a search for "python projects for beginners." You'll quickly see a wide array of suggestions, from games to weather tracking to gathering information on stocks.
Common Concepts
Python shares features with other programming languages. After all, developers need to solve similar problems using applications, so the concepts are fairly standard. Below are a few examples of concepts Python uses.
Indention
Python uses indentation to define code blocks. The quantity of indentations (spaces) does not matter, though it must be consistent throughout the application. Most Python developers use four spaces by convention, so that's probably a good habit to get into.
Comments
Developers use comments in code to provide explanations for code blocks, offer suggestions or examples, or call out problematic sections with warnings. Most programming languages have specific characters to designate a comment. In Python, that character is #.
Variables and Data Types
Variables store strings of values for use later by the application or script. Python does not have a specific command for declaring variables; the values are simply set the first time the variable is defined.
Operators
Operators perform various operations on Python data (variables and values). These may include comparisons and arithmetic operations to generate results from collected data.
Control Structures
Python uses control structures to control other blocks of code. These blocks might include checking conditions using if-else statements, looping sections of code for a specific number of times or while a condition exists and calling functions (blocks of code called when needed by other blocks of code).
Get Started With Python
Now you have a good idea of what Python is and why you might want to learn it. It's time to get started. First, install the latest Python package on your system of choice (Linux, macOS or Windows). Confirm the installation by running a few commands, and then find a good project online to start on.
Install Python on Linux
Use your distribution's package manager to install Python. Linux releases, including various older versions, are available from the Python site in a Linux-specific listing.
On Ubuntu or similar, type:
sudo apt-get update
sudo apt-get install python3.10
On Red Hat or similar, type:
sudo dnf install python3
Note that depending on your distribution, you may need to add a repository to your package manager. Also, many Linux distributions include Python.
Install Python on macOS
Depending on your macOS version, you may already have Python 2.7 installed. However, recall that the 2.7 version is retired, so you'll likely want to update to Python 3. Download the Python package for macOS from the Python site. Follow the standard installation instructions to add Python3 to your Mac.
Install Python on Windows
Windows users can download 32-bit and 64-bit installers from the Windows portion of the Python site. Double-click the installer and follow the wizard to complete the installation.
Confirm the installation
Once you've completed the installation, run the python3 --versions command from the terminal to confirm the installation. The following screenshot shows Python 3.8.2 on my system:
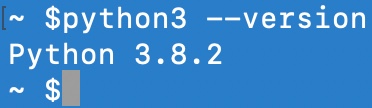
Figure 4: Python3 version 3.8.2 installed on this system
Now you're ready to run your first Python commands. Open a terminal, enter the Python environment, and print the "Hello, World!" message to your screen. Here's an example:
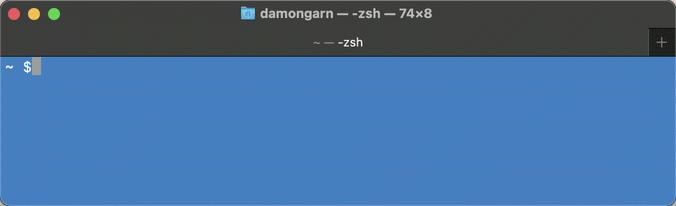
Figure 5: Running a Hello World test using Python3
The specific commands are:
python3
>>> print('Hello, World!')
>>> quit()
Next, search the web and select a beginner project that interests you. There are plenty of ideas out there to get you started.
The example above demonstrates running Python commands directly in the Python environment in a command-line interface. This is useful in some cases but is a manual process that is difficult to repeat frequently. Save your Python code in a file with a .py extension and then run the file with the python3 command to execute a larger application.
Python Versus Other Programming Languages
Most programming languages share similar concepts, such as variables, functions, operators, etc. However, each differs by syntax and use. For example, JavaScript is used for both front- and back-end web development, whereas Python is typically back-end only. C++ and Java (not to be confused with JavaScript) are also Python competitors. C++ and Java are compiled languages, making them faster than Python. However, they are also more complex and difficult to learn. C++ and Java are often used for larger enterprise applications where scalability is valued. And while Python has a limited ability to query databases, languages such as SQL (Structure Query Language) are designed to pull information more efficiently and easily.
It's worth noting that the 2023 Stack Overflow Developer Survey ranks Python as the third most popular language (up from fourth place in 2022). JavaScript remains at the head of the pack, with HTML/CSS in second place. While that might tempt some readers into selecting JavaScript over Python to get started, recall that choosing a language should be oriented on your goals or the project requirements rather than popularity.
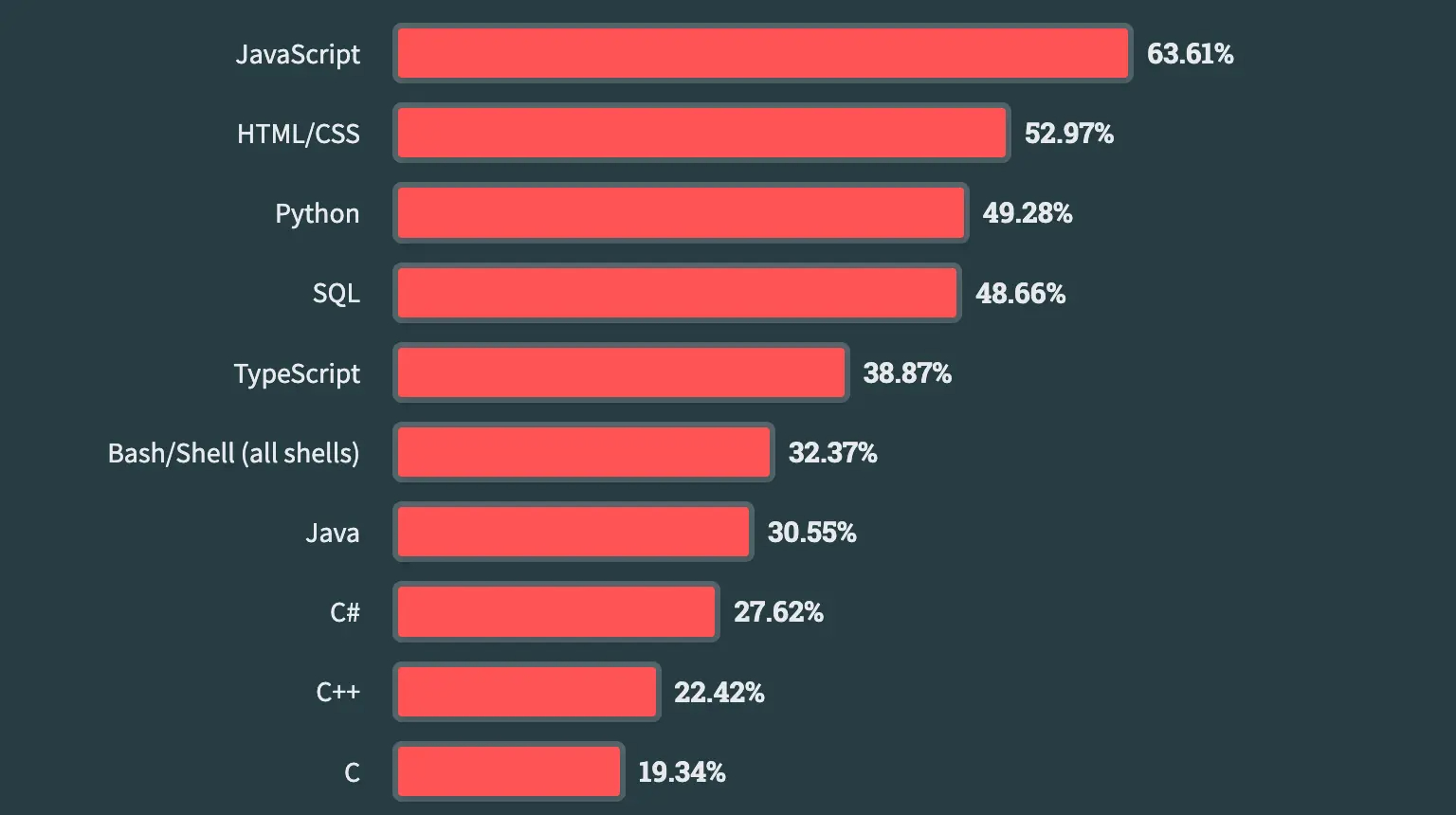
Figure 6: 2023 Stack Overflow Developer Survey of programming languages
Python Advantages and Disadvantages
Here are a few general observations about Python to help you determine whether to use it.
Python Advantages:
- Maintains a very large support community
- Extensive collection of libraries
- Easy to learn and code
- Easy to read for interpretation and debugging
- Cross-platform (Linux, macOS, Windows)
- Free and open source
Python Disadvantages:
- Runs slower than other languages, such as C++ or Java
- Not a good solution for mobile application development
- Does not handle memory-intensive solutions efficiently
- Some developers miss the features of Python and find it difficult to learn other programming languages
Python Is Popular for a Reason
With Python's functionality in machine learning, data analysis and artificial intelligence, its future is well-assured. Python keeps rising in popularity among developers, and the industries where it makes the biggest difference continue to grow. Python's simplicity makes it attractive to experienced developers and those new to the field, and one cannot downplay the importance and involvement of its user community.
Because Python is not a traditionally compiled programming language and with its speed and scalability limitations, there is sometimes a misconception by some developers that Python is not a "real" programming language. However, its use and popularity certainly cannot be ignored.
This article provides enough information for you to get started today with Python. Download the latest Python version for your Linux, Mac or Windows system (or Raspberry Pi!) and run the installer. Then browse the web for a beginner project that looks interesting or useful to you, and progress from there. And maybe turn on an old Monty Python episode in the background, too.
Ready to upgrade your IT skills? We've got great news! You can save big on CompTIA certifications and training right now.